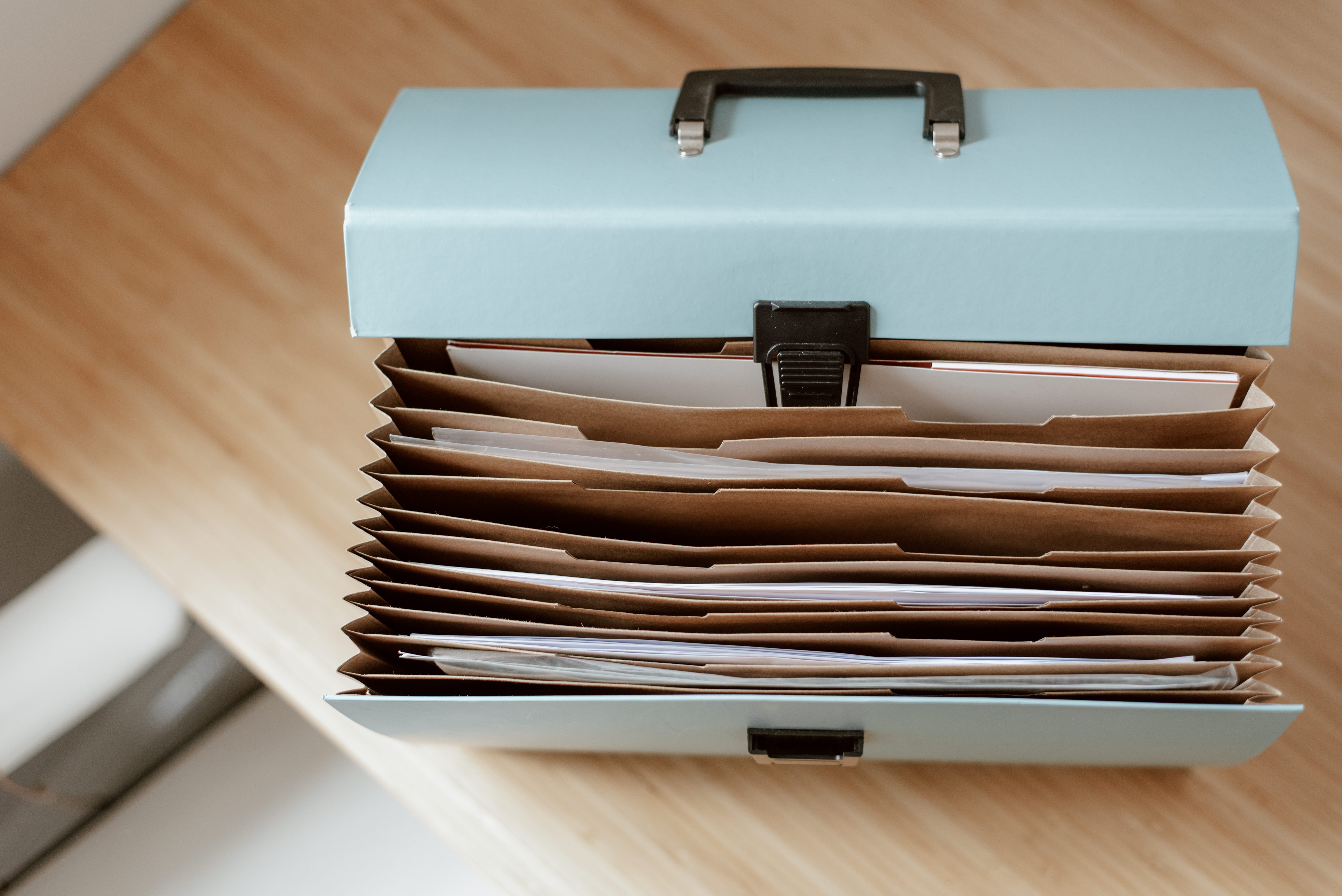
Why
It seems that for most of my life I have been dealing with a quite messy downloads folder. I try to organize it by moving some of the things outside of it. Sometimes I even delete all its contents. Most of the time though, I end up leaving quite a lot of files in there as I realize I might need those later but I have nowhere else to put them.
This is quite a common problem of mine and I decided to look for a solution. I tried looking for some kind of application that manages my downloads or that will organize them into different categories, but this not only implies that I’ll have to use a different application apart from my default file manager, but it may not work on all my devices as it would require a cross-platform application, or searching for a different application for each platform.
This is how I found that an easy way of doing it is using a Python script. This will not only allow me to customize the file organization according to my needs but it will also work on all platforms that support Python (Linux, Windows, MacOS).
How
Create a categorization system
We will use the enum module to create our own categorization system. Each enum will correspond to a folder inside of which we define the file types that will be added. We will do this by bundling types of file extensions.
|
|
In this example, we have defined 5 categories, but you can go ahead and customize them however you like. On top of these five, we will also add an empty category named ‘Others’ that will provide a folder for the unknown types of files that we may encounter.
Now that we have defined the folders, we will add all the enums in a list that we can easily iterate through.
Find and move the files
The most important function of the script is organize_files(). In this function we search the directory located at the path provided as a function argument and add all the found files to the list “files”. This will skip over the folders that are located at this location so it will not mess with the already organized files that you have there. Only the files outside of a folder will be affected.
|
|
Once we get all the files, we iterate through the file list and take action for each file.
- First we take the file extension by splitting the file name using ‘.’ as a delimiter and taking the last string.
- Now that we have our file extension we iterate through our enum list that we have created earlier and see in which folder our file will be moved. If we iterate through the entire list and don’t manage to find a folder, we use our last enum defined in this list, in our case being Others that we have specifically defined for this scenario.
- Before moving our file to the destination path, we must verify if the folder already exists and create it in case it doesn’t. An error will occur if we try to move our file to a path that doesn’t exist and our script will close.
- Finally, we move the file to the destination path using the move function from the shutil module.
Make it cross platform
As we set our goal to make this script work on multiple platforms we need to make sure it works regardless of the operating system it’s running on. While the Python runtime can be installed on all three major platforms (Linux, Windows, MacOS), the way each operating system encodes paths is different. Not only is the format different but they also have different file system hierarchies that we need to account for.
We first need to find the Downloads folder path on each platform:
|
|
We use the sys.platform function to find the operating system we are running on and define our path accordingly.
Now that we have the paths we need to get the user that launches this script, as most of the times this is where our target folder will be. We do this by using the get getuser function from the getpass module and replace the {USER} string in the path with the result of the function.
For Windows, the paths are written using ‘\’ instead of ‘/’. The backslash is used in most programming languages as an escape character. This means that it is a special character that our program will interpret differently and it will not show in our string. For more information search for escaping character, but the gist of it is that we use a backslash before a special character to specify that we want the special character to be interpreted litteraly.
To solve this problem we can use another backslash to escape the second one like this:
|
|
Or we can define the string as a raw string so the characters will be interpreted as they are written:
|
|
Both methods will work the same. For this script I have chosen the first one.
Add command line argument
We now have a complete script that will do exactly what we want and it will work on all platforms. But I still think it needs a little addition to make it more versatile.
We have defined a default way in which our script can find a correct path to work in. These are fine most of the time, but sometimes we may have a different directory the we want to organize and we need to cover that aspect as well.
We make the script to check for command line arguments. If an argument is provided it will use that as the path, otherwise it will go and try to find that path on its own using the get_path() function we have created above.
|
|
We check for command line arguments using the system.argv variable. It is a list containing all the arguments given when the script is called.
For example, if we call $ python main.py a
the arguments that are stored in the argv list are: ['main.py','a']
This means that the second element in the list is the one we are looking for. If there is only one element in the list it means no argument has been provided.
Automate the script trigger
Now that our script is final we need to call it. We can do so using:
|
|
Or
|
|
This is quite simple, but it will get more complicated when the working directory is not the same as the directory where we keep the script. To make the process of calling it easier we will explore two solutions. It is up to you to decide which one you think will work best for you.
Bash alias
The first solution is to simply create a bash alias that will call our script. This is a quick and simple solution. You can call the script easier from anywhere, but you will have to do it manually every time and it will only work on Linux (MacOS should work as well, but I cannot test it).
All you have to do is add the following line to your .bashrc file and then log out and log back in. Make sure to replace the absolute path to your python script.
|
|
This way you can call your script by simply opening a terminal and typing:
|
|
If you need more information I sugest searching how to create an alias on your working environment.
Cronjobs and Task Scheduler
The second solution, and the more elegant one, is to schedule a task that will be triggered at a certain time. This is a bit more complicated than setting a bash alias, but it will provide a seamless integration with your work flow. This will work on all platforms as well.
- If you are looking for a solution on Linux or MacOS you should checkout how to setup a cronjob.
- If you are on Windows create a batch file that inside of which you call the python script. Then look for scheduling the execution of this batch fiel using the windows task scheduler.
Conclusion
Our file organizing solution is finished. Using this script you can keep your downloads folder completely organized and make sure you can easily find the file you are looking for.
As an added bonus, the newly downloaded files are always outside the organized folders so that way you can easily see them without searching too much and after you run the script they will be categorized and moved to their own respective folder.
If you want to take a look at the source code you can find it on Github. If you liked it please give it a star or support me directly.